Introduction to the HelloPogo Chat Widget
The HelloPogo Chat Widget is an AI-powered assistant that seamlessly integrates with your website to provide instant help to your users based on your automatically generated documentation. Once implemented, the widget will:
- Answer user questions about your product features and workflows
- Guide users through complex processes
- Reduce support tickets by providing immediate assistance
- Continuously improve as your documentation evolves
AI-Powered Assistance
Trained on your specific documentation to provide accurate, contextual help.
Customizable Interface
Match your brand colors, positioning, and messaging for a seamless experience.
Real-Time Updates
Automatically stays current with your latest documentation changes.
Preview of the HelloPogo Chat Widget
Implementation Steps
Follow these simple steps to add the HelloPogo Chat Widget to your website:
Include the Widget Script
Add the Pogo Chat Widget script to your website by placing this code just before the closing </body> tag:
<script src="assets/js/pogo-chat-widget.js"></script>
(Optional) Configure the Widget
For custom configuration, add this script before the widget script:
<script>
window.PogoChatConfig = {
baseUrl: 'http://localhost:3000',
projectId: '67f092a01f1e60f7ed5e4724',
userId: 'yash@highscores.ai',
logo: 'assets/img/hellopogo-logo.webp',
widgetTitle: 'HelloPogo Assistant',
chatIcon: 'assets/img/pogo-chat.webp'
};
</script>
Test the Widget
After adding the scripts, refresh your website to verify the chat widget appears. It should display as a chat icon in the corner of your page.
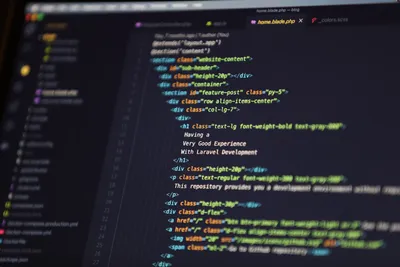
The widget comes with these default settings if you don't specify your own:
- Web Server: https://ai.hellopogo.com
- Project ID: 67f092a01f1e60f7ed5e4724
- User ID: yash@highscores.ai
Make sure to update these values for your production environment.
Customization Options
The HelloPogo Chat Widget can be customized to match your brand and website design. Here are the available customization options:
Brand Colors
The widget uses these brand colors by default:
- Primary: #144464
- Secondary: #7cdc54
- Accent: #0494f3
- Background: #dce8e1
- Text: #247cb4
- Border: #94acbc
You can customize these colors by adding them to your configuration:
<script>
window.PogoChatConfig = {
baseUrl: 'https://ai.hellopogo.com/',
projectId: 'your-project-id',
userId: 'your-email-id',
// Color customization
colors: {
primary: '#144464',
secondary: '#7cdc54',
accent: '#0494f3',
background: '#dce8e1',
text: '#247cb4',
border: '#94acbc'
}
};
</script>
Widget Features
The HelloPogo Chat Widget includes these built-in features:
- Real-time chat interface
- Automatic session management
- Chat history support
- Typing indicators
- Responsive design
- Customizable colors and settings
Advanced Configuration
For more advanced customization, you can configure additional options:
<script>
window.PogoChatConfig = {
baseUrl: 'https://ai.hellopogo.com/',
projectId: 'your-project-id',
userId: 'your-email-id',
// Widget appearance
position: 'bottom-right', // Widget position (bottom-right, bottom-left, top-right, top-left)
initialMessage: 'Hi! How can I help you today?', // First message from the bot
widgetTitle: 'Product Support', // Title displayed in the widget header
// Widget behavior
autoOpen: false, // Whether to automatically open the widget
displayDelay: 3000, // Delay before showing widget (in ms)
persistSession: true, // Save chat history between page loads
enableTypingIndicator: true, // Show typing indicators
// Advanced options
allowAttachments: false, // Enable file attachments
enableVoiceInput: false, // Enable voice input option
maxHistoryItems: 50 // Max number of messages to store in history
};
</script>
For a complete list of customization options, see our Advanced Customization Documentation.
Advanced Implementation
For websites built with modern JavaScript frameworks, you can still use the basic implementation method, but you may need to adjust when the script loads:
React Implementation
For React applications, you can add the chat widget in your main component:
import React, { useEffect } from 'react';
// Chat widget configuration component
function PogoChatWidget() {
useEffect(() => {
// Set global config
window.PogoChatConfig = {
baseUrl: 'https://ai.hellopogo.com/',
projectId: 'your-project-id',
userId: 'your-email-id'
};
// Load the widget script
const script = document.createElement('script');
script.src = 'assets/js/pogo-chat-widget.js';
script.async = true;
document.body.appendChild(script);
// Cleanup on unmount
return () => {
document.body.removeChild(script);
};
}, []);
return null; // This component doesn't render anything
}
// Use in your App or layout component
function App() {
return (
{/* Your app content */}
);
}
export default App;
Angular Implementation
For Angular applications, create a service and component to load the widget:
// chat-widget.service.ts
import { Injectable, Inject } from '@angular/core';
import { DOCUMENT } from '@angular/common';
@Injectable({
providedIn: 'root'
})
export class ChatWidgetService {
private loaded = false;
constructor(@Inject(DOCUMENT) private document: Document) {}
loadWidget() {
if (this.loaded) return;
// Set configuration
(window as any).PogoChatConfig = {
baseUrl: 'https://ai.hellopogo.com/',
projectId: 'your-project-id',
userId: 'your-email-id'
};
// Create and load script
const script = this.document.createElement('script');
script.src = 'assets/js/pogo-chat-widget.js';
script.async = true;
this.document.body.appendChild(script);
this.loaded = true;
}
}
// chat-widget.component.ts
import { Component, OnInit } from '@angular/core';
import { ChatWidgetService } from './chat-widget.service';
@Component({
selector: 'app-chat-widget',
template: ''
})
export class ChatWidgetComponent implements OnInit {
constructor(private chatWidgetService: ChatWidgetService) {}
ngOnInit() {
this.chatWidgetService.loadWidget();
}
}
// Add this component to your app.component.html template
Vue Implementation
For Vue applications, create a component to load the chat widget:
// ChatWidget.vue
<template>
<!-- Component doesn't render anything -->
</template>
<script>
export default {
name: 'ChatWidget',
mounted() {
// Set configuration
window.PogoChatConfig = {
baseUrl: 'https://ai.hellopogo.com/',
projectId: 'your-project-id',
userId: 'your-email-id'
};
// Create and load script
const script = document.createElement('script');
script.src = 'assets/js/pogo-chat-widget.js';
script.async = true;
document.body.appendChild(script);
},
beforeUnmount() {
// Find and remove the script
const scripts = document.getElementsByTagName('script');
for (let i = 0; i < scripts.length; i++) {
if (scripts[i].src.includes('pogo-chat-widget.js')) {
document.body.removeChild(scripts[i]);
break;
}
}
}
}
</script>
<template>
<div id="app">
<!-- Your app content -->
<ChatWidget />
</div>
</template>
<script>
import ChatWidget from './components/ChatWidget.vue'
export default {
components: {
ChatWidget
}
}
</script>
WordPress Implementation
For WordPress sites, add the widget code to your theme's footer.php file or use a plugin:
<?php
/**
* The template for displaying the footer
*/
?>
<!-- Your footer content -->
<!-- Pogo Chat Widget -->
<script>
window.PogoChatConfig = {
baseUrl: 'https://ai.hellopogo.com/',
projectId: 'your-project-id',
userId: 'your-email-id'
};
</script>
<script src="assets/js/pogo-chat-widget.js"></script>
</body>
</html>
Alternatively, you can use a custom WordPress plugin or a "Custom HTML" block in your footer widget area to add the code.
Widget Analytics & Features
The Pogo Chat Widget includes several powerful features to help your users get the most out of your documentation:
Built-in Features
- Real-time chat interface - Provides a familiar messaging experience
- Automatic session management - Handles user sessions without additional code
- Chat history support - Preserves conversations across page refreshes
- Typing indicators - Shows when the AI is preparing a response
- Responsive design - Works seamlessly on desktop and mobile devices
- Customizable interface - Adapts to your brand and website design
Analytics Dashboard
You can access widget analytics through your HelloPogo dashboard to gain insights about user interactions:
- Log in to your HelloPogo account
- Navigate to your project
- Select the "Analytics" tab
Available Metrics
The analytics dashboard provides valuable information such as:
- Usage Statistics: Total conversations, messages, and active users
- Common Questions: Most frequently asked questions by your users
- Response Quality: How well the AI is addressing user queries
- User Engagement: Average conversation length and engagement metrics
- Peak Usage Times: When your widget receives the most traffic
Custom Event Tracking
You can track specific events and user interactions by adding custom tracking code:
<script>
// Set up event listener after widget loads
document.addEventListener('pogoChatReady', function() {
// Track when a user starts a new conversation
window.PogoChat.on('conversationStart', function(data) {
console.log('New conversation started', data);
// You can send this to your analytics system
});
// Track when a user receives an answer
window.PogoChat.on('messageSent', function(data) {
console.log('Message sent', data);
});
// Track when a user closes the chat
window.PogoChat.on('widgetClosed', function() {
console.log('Chat widget closed');
});
});
</script>
Best Practices
Follow these recommendations to get the most out of your Pogo Chat Widget implementation:
Implementation Best Practices
- Place script at the end: Always add the widget script just before the closing </body> tag to ensure your page loads properly.
- Use production URLs: For production environments, always use the full https://www.hellopogo.com domain, not localhost URLs.
- Test thoroughly: Test your widget on multiple devices and browsers to ensure consistent functionality.
- Set up proper project IDs: Use different project IDs for development and production environments.
Content Optimization
- Complete documentation: Record comprehensive documentation sessions to train your AI assistant.
- Focus on common workflows: Prioritize documenting the most commonly used features and workflows.
- Update regularly: Re-record documentation when your interface changes to keep the AI knowledge current.
- Use clear descriptions: When recording with the Chrome extension, provide clear, concise verbal explanations.
User Experience
- Set clear expectations: Configure a welcoming message that explains what the chat widget can help with.
- Brand consistency: Customize colors and styling to match your website's design.
- Mobile optimization: Test and adjust the widget position on mobile devices for optimal experience.
- Don't hide important content: Ensure the widget doesn't obstruct important page elements.
Frequently Asked Questions
// Example: Only show widget on product pages
if (window.location.pathname.includes('/products')) {
// Add the Pogo Chat Widget script
}
Need Additional Help?
If you're experiencing issues with your widget implementation or have questions not covered in this guide, our support team is here to help:
- Email us at support@hellopogo.com
- Chat with us using the support widget on our website
- Visit our help center for more resources
- Schedule a technical consultation for custom implementation assistance